- Published on
What is an Application Programming Interface (API)?
- Authors
- Name
- codewithininsight
- @
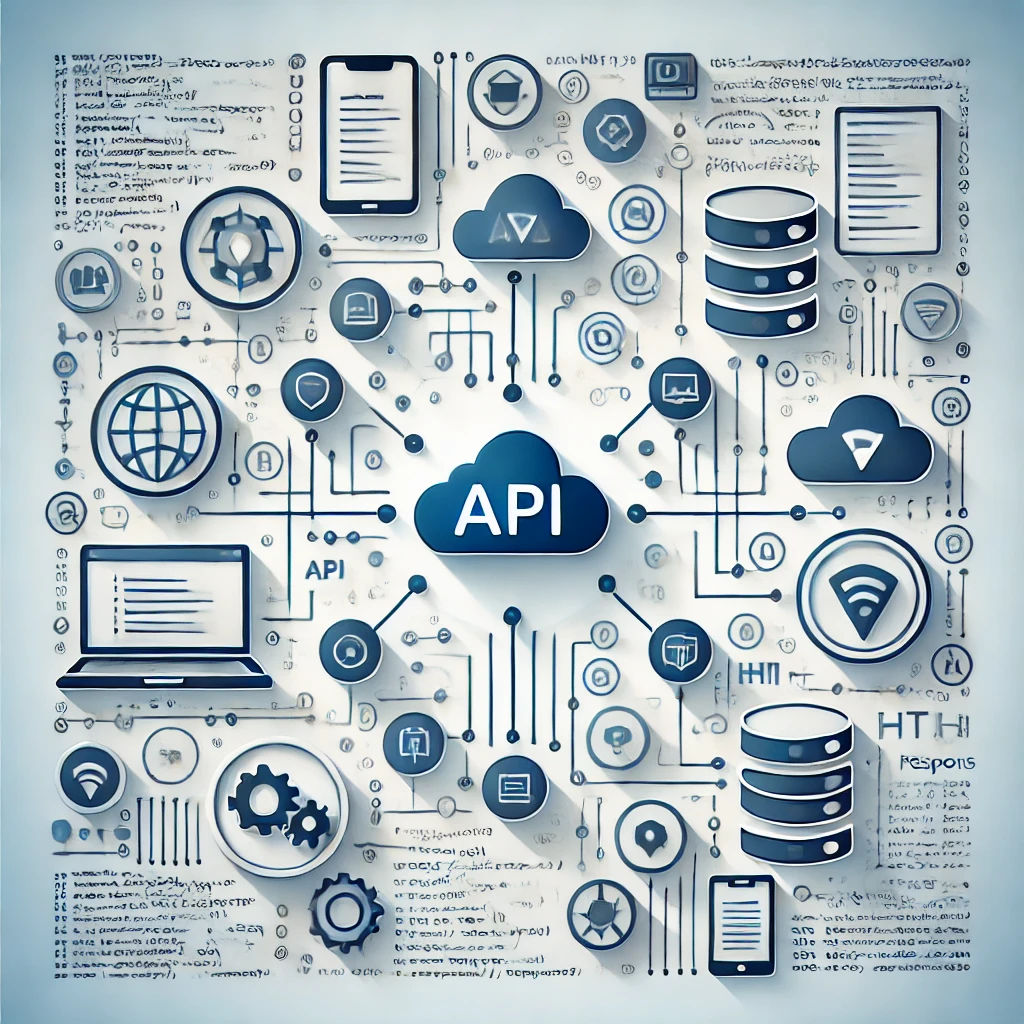
In this blog, we’ll explore what an Application Programming Interface (API) is, how it works, and its significance in the world of software development.
What is an API?
An Application Programming Interface (API) is a set of rules and protocols that allows different software applications to communicate with each other. Think of it as a bridge that enables two systems to interact without needing to know how the other works internally.
Simple Analogy:
Imagine you’re at a restaurant. The menu is like the API—it defines what you can order (the services or data you can request). The waiter acts as the intermediary, taking your request (an API call) to the kitchen (the system) and delivering your food (the response) back to you.
How Does an API Work?
An API works through requests and responses:
- Client Request: A user or application sends a request to the API, specifying the desired data or action.
- Server Processing: The server processes the request and retrieves or manipulates the necessary data.
- Response: The API sends the processed data back to the client.
Example: Fetching Weather Data
If you want to display weather information in your app:
- Your app makes a GET request to a weather API like
api.weather.com
. - The API returns data like temperature, humidity, and wind speed, which your app can display.
Types of APIs
APIs come in various forms, depending on their use case:
Web APIs (HTTP APIs):
These are accessed over the internet and are the most common. Examples include RESTful APIs and GraphQL APIs.Library APIs:
Provided by programming libraries, they allow you to interact with specific functions or services within the library.Operating System APIs:
Allow apps to interact with the operating system, like file systems or device hardware.Third-Party APIs:
Provided by external services like Twitter API, Google Maps API, or Stripe API for integrations.
Why Are APIs Important?
APIs are fundamental to modern software development for several reasons:
- Integration: APIs enable seamless integration between different applications and services.
- Scalability: They allow systems to grow and evolve independently.
- Efficiency: Developers can reuse existing APIs to save time instead of building from scratch.
- Innovation: APIs empower developers to create new tools, products, and integrations.
Real-World Examples of APIs
Here are some popular APIs you’ve likely encountered:
- Google Maps API: Used by apps to embed maps and navigation.
- Twitter API: Allows developers to fetch tweets or automate interactions.
- Stripe API: Enables secure payment processing for e-commerce platforms.
- Spotify API: Lets developers integrate music playback and data.
Common API Protocols
APIs use various protocols for communication. The most common ones are:
REST (Representational State Transfer):
- Relies on HTTP methods like GET, POST, PUT, DELETE.
- Example: Fetching user data with
GET /users
.
GraphQL:
- Allows clients to request specific data they need.
- Example: Querying user name and email in one request.
SOAP (Simple Object Access Protocol):
- An older, more structured protocol often used in enterprise systems.
How to Use an API
Here’s a simple example of making a GET request to an API using JavaScript:
async function fetchData() {
const response = await fetch('https://api.example.com/data')
const data = await response.json()
console.log(data)
}
fetchData()
Challenges with APIs
While APIs are powerful, they come with challenges:
- Rate Limits: APIs often restrict the number of requests you can make in a given time.
- Security: Exposing an API requires careful handling of sensitive data.
- Versioning: Updating APIs can break older integrations if not managed properly.
Conclusion
An Application Programming Interface (API) is a vital tool in software development, enabling seamless communication between applications and systems. Whether you're fetching weather data, processing payments, or embedding maps, APIs are at the heart of modern technology.
Understanding how APIs work opens up countless possibilities for building integrations, improving functionality, and creating innovative solutions